Be sure to read all of this document carefully, and follow the guidelines within.
-
We use fvm for managing the flutter version within the project. Using terminal, while being on the test repository, install the tools dependencies by running the following commands:
dart pub global activate fvm
The output of the command will ask to add the folder
./pub-cache/bin
to your PATH variables, if you didn't already. If that is the case, add it to your environment variables, and restart the terminal.export PATH="$PATH":"$HOME/.pub-cache/bin" # Add this to your environment variables
-
Install the project's flutter version using
fvm
.fvm use
-
From now on, you will run all the flutter commands with the
fvm
prefix. Get all the projects dependencies.fvm flutter pub get
More information on the approach can be found here:
hhttps://fvm.app/docs/getting_started/installation
From the root directory:
Use with VSCode
If you're a VScode user link the new Flutter SDK path in your settings
$projectRoot/.vscode/settings.json
(create if it doesn't exist yet)
{
"dart.flutterSdkPath": ".fvm/flutter_sdk"
}
Use with IntelliJ / Android Studio
Go to Preferences > Languages & Frameworks > Flutter
and set the Flutter SDK path to $projectRoot/.fvm/flutter_sdk
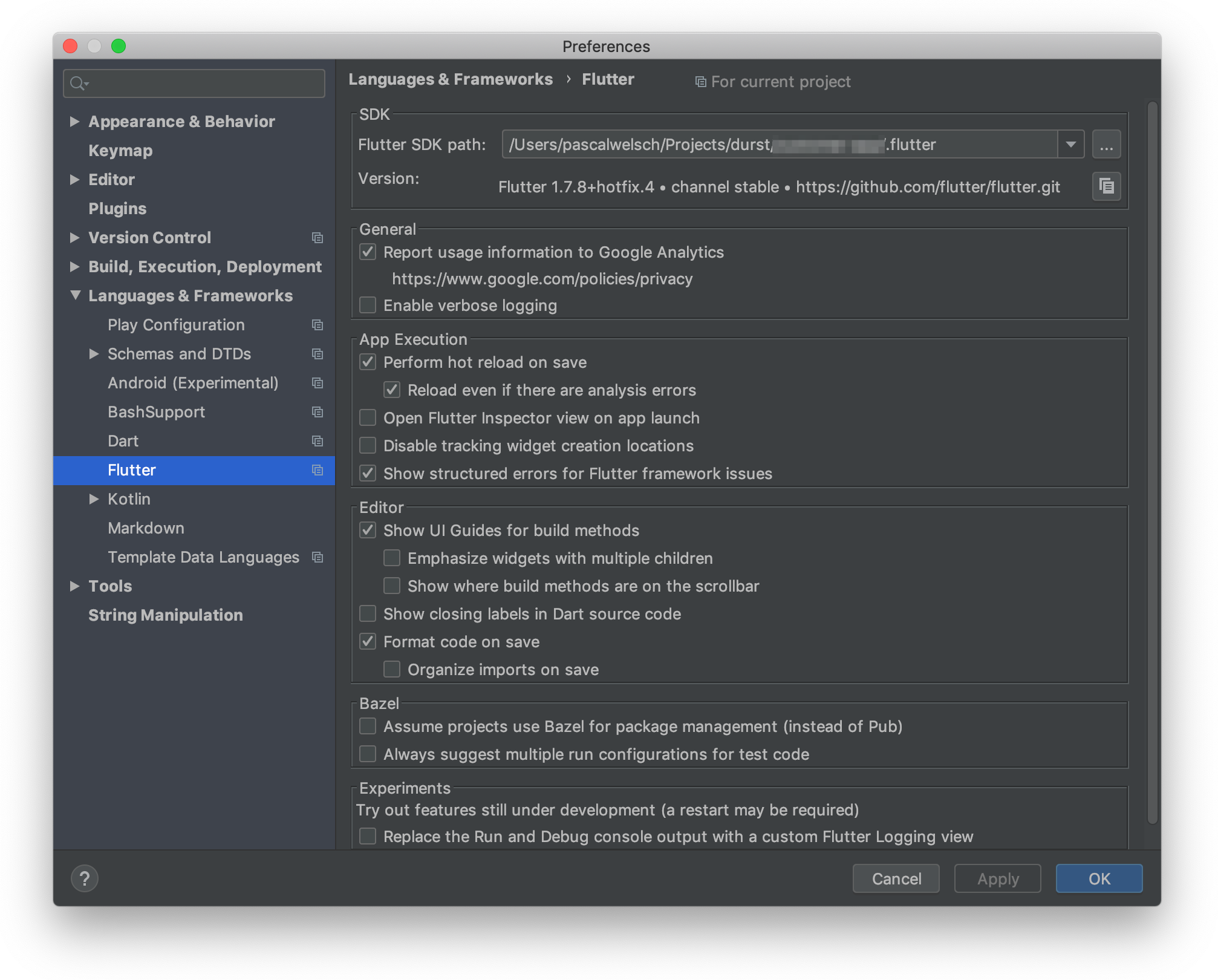
- Tab Bar
- List of favorites (stored client side)
- List of businesses
- Hero image
- Name
- Price
- Category
- Rating (rounded to the nearest value)
- Open/Closed
- Ability to favorite a business
- Name
- Hero image
- Price and category
- Address
- Rating
- Total reviews
- List of reviews
- User name
- Rating
- User image
- Review Text (These are just snippets of the full review, usually like 3-4 lines long)
- Clear documentation on the structure and architecture of your application.
- Clear and logical commit messages.
- We suggest following Conventional Commits
To demonstrate your experience writing different types of tests in Flutter please do the following:
- Choose ONE portion of your state management and write a unit test.
- Choose ONE widget and write a widget test.
Feel free to add more tests as you see fit but the above is the minimum requirement.
- See this Figma File for design information related to the overall look and feel of the application. We do not expect pixel-perfection but would like the application to visually be close to what is specified in the Figma file.
The Yelp GraphQL API is used as the API for this Application. We have provided the boilerplate of the API requests and backing data models to save you some time. To successfully make a request to the Yelp GraphQL API, please follow these steps:
- Please go to https://www.yelp.com/signup and sign up for a developer account.
- Once signed up, navigate to https://www.yelp.com/developers/v3/manage_app.
- Create a new app by filling out the required information.
- Once your app is created, scroll down and join the
Developer Beta
. This allows you to use the GraphQL API. - Copy your API Key from your app page and paste it on
line 5
yelp_repository.dart replacing the<PUT YOUR API KEY HERE>
with your key. - Run the app and tap the
Fetch Restaurants
button. If you see a log likeFetched x restaurants
you are all set!
Please restrict your usage of state management or dependency injection to the following options:
We ask this because this challenge values consistency and efficiency over ingenuity. Using commonly used libraries ensures that we can review your code in a timely manner and allows us to provide better feedback.
At Superformula we strive to build applications that have
- Consistent architecture
- Extensible, clean code
- Solid testing
- Good security & performance best practices
Approach your submission as if it were a real world app. This includes Use any libraries that you would normally choose.
Please note: we're interested in your code & the way you solve the problem, not how well you can use a particular library or feature.
Writing boring code that is easy to follow is essential at Superformula.
We're interested in your method and how you approach the problem just as much as we're interested in the end result.
While the purpose of this challenge is not to gauge whether you can achieve 100% test coverage, we do seek to evaluate whether you know how & what to test.
Where should I send back the result when I'm done?
Please fork this repo and then send us a pull request to our repo when you think you are done. There is no deadline for this task unless otherwise noted to you directly.
What if I have a question?
Just create a new issue in this repo and we will respond and get back to you quickly.
The coding challenge is a take-home test upon which we'll be conducting a thorough code review once complete. The review will consist of meeting some more of our mobile engineers and giving a review of the solution you have designed. Please be prepared to share your screen and run/demo the application to the group. During this process, the engineers will be asking questions.