diff --git a/content/adventures/zun.yaml b/content/adventures/zun.yaml
new file mode 100644
index 00000000000..39182e98d7c
--- /dev/null
+++ b/content/adventures/zun.yaml
@@ -0,0 +1,1094 @@
+adventures:
+ songs:
+ default_save_name: Song
+ levels:
+ 6:
+ story_text_2: "This children's song counts down from 5 little monkeys to 1 monkey.\nIf you copy line 2 - 7 and paste it under the the code, you can sing the whole song!\n"
+ example_code_2: "```\nnumber = 6\nnumber = number - 1\n{print} number ' little monkeys jumping on the bed'\n{print} 'One fell off and bumped his head'\n{print} 'Mama called the doctor and the doctor said'\n{print} 'NO MORE MONKEYS JUMPING ON THE BED!'\n{sleep}\n```\n"
+ example_code: "```\nverse = 99\n{print} verse ' bottles of beer on the wall'\n{print} verse ' bottles of beer'\n{print} 'Take one down, pass it around'\nverse = verse - 1\n{print} verse ' bottles of beer on the wall'\n{sleep}\n```\n"
+ story_text: "Songs often contain a lot of repetition. Sometimes the repetition is also based on counting.\nFor example, in the well-known song 'Bottles of beer'. You can program that song with a little math.\n\nTip: Use the read aloud function to let Hedy sing the song to you!\n\n### Exercise\nYou can now repeat lines 2 to 7 as many times as you want by copying the lines.\n"
+ 7:
+ example_code: "```\n{repeat} _ _ {print} 'Baby Shark tututudutudu'\n{print} 'Baby Shark'\n```\n"
+ story_text: "Songs often contain a lot of repetition. For example... Baby Shark! If you sing it, you keep singing the same thing:\n\nBaby Shark tututudutudu
\nBaby Shark tututudutudu
\nBaby Shark tututudutudu
\nBaby Shark\n\n### Exercise\nYou can print the song Baby Shark with a `{repeat}`? Finish the code by replacing the blanks?\n**Extra** After Baby Shark you can of course also program other songs. There are many songs with repetition!\nCan you think of one more song and print it?\n"
+ 10:
+ example_code_2: "```\nmonkeys = 5, 4, 3, 2, 1\n```\n"
+ example_code: "```\nfamily = baby, mammy, daddy, grandma, grandpa\n_ _ _ _ \n {print} _\n```\n"
+ story_text: "With `{for}` you can print make the whole baby shark song (including all the other sharks in the family) in only 6 lines!\n\n### Exercise 1\nCan you make the baby shark code even shorter by using a `{for}` command? Finish the example code.\n"
+ story_text_2: "### Exercise 2\nPrint the song Five little moneys jumping on the bed. Look up the text if you don't remember.\n\n**Extra** Print the song Old MacDonald had a farm, and make sure all animals make a different sound, using an `{if}`.\n"
+ 11:
+ example_code: "```\n_ monkeys _ _ 5 _ 1\n {print} monkeys ' little monkeys jumping on the bed'\n _\n```\n"
+ story_text: "In this level you can use the `{for}` with `{range}` to make songs that use counting, like the 5 little monkeys.\n\n### Exercise 1\nFill in the blanks and make the code work! If you don't remember the song text, look it up yourself.\n\n### Exercise 2\nThe final line of the song is different from the others. Print this line inside the `{for}`, and use an `{if}` to make it work correctly.\n"
+ 18:
+ example_code: "```\nlines = ['what shall we do with the drunken sailor', 'shave his belly with a rusty razor', 'put him in a long boat till hes sober']\n{for} line {in} lines _\n {for} i {in} {range} 1 {to} 3 _\n {print} _ line _\n {print} 'early in the morning'\n {for} i {in} {range} 1 {to} 3\n {print} 'way hay and up she rises'\n {print} 'early in the morning'\n```\n"
+ story_text: "In level 16 we made songs using lists. These programs however are no longer working properly in this level. The colons from level 17 and the brackets from level 18 still need to be added.\n\n### Exercise 1\nThe Drunken sailor song is given as sample code, but not yet working.\nCan you make sure everything works again? To help you, we've put _ in the places of _some_ errors.\n\n### Exercise 2\nNow also look up your Old MacDonald song from level 16, and correct it.\n"
+ 8:
+ example_code: "```\nverse = 99\n_ 99 {times}\n{print} verse ' bottles of beer on the wall'\n{print} verse ' bottles of beer'\n{print} 'Take one down, pass it around'\nverse = verse - 1\n{print} verse ' bottles of beer on the wall'\n```\n"
+ story_text: "In a previous level you've programmed the song 'Bottles of beer'. But without the `{repeat}` command, you had to copy the verses many times.\nIn this level you can repeat the song 99 times, just by adding one simple line!\n\n### Exercise\nAdd the right command on the blanks and indent the code correctly.\n"
+ 13:
+ story_text: "In the previous adventure you have learned how to use an argument in a function, but did you know that you could combine them with {ask} commands as well?\nIn this example we have changed the 'My Bonnie' program and made it interactive. You are now asked where Bonnie is.\n"
+ example_code: "```\n{define} song {with} place\n {print} 'My Bonnie is ' place\n\nchosen_place = {ask} 'Where do you want Bonnie to be?'\nsynonym = {ask} 'What is another word for that?'\n\n{call} song {with} chosen_place\n{call} song {with} synonym\n{call} song {with} chosen_place\n```\n"
+ 16:
+ story_text: "In this level, you can program a song like OldMacDonald even more quickly. You can connect the right animal to the right sound by simply putting them in the same place in the list.\nThe Drunken Sailor is also quickly made in this level. You only need 8 lines for the entire song, check it out!\n\n### Exercise\nComplete the Old MacDonald song by setting the variable `animal` to `animals[i]` and `sound` to `sounds[i]`.\n"
+ example_code: "```\nanimals = ['pig', 'dog', 'cow']\nsounds = ['oink', 'woof', 'moo']\n{for} i {in} {range} 1 {to} 3\n animal = _\n sound = _\n {print} 'Old MacDonald had a farm'\n {print} 'E I E I O!'\n {print} 'and on that farm he had a ' animal\n {print} 'E I E I O!'\n {print} 'with a ' sound sound ' here'\n {print} 'and a ' sound sound ' there'\n {print} 'here a ' sound\n {print} 'there a ' sound\n {print} 'everywhere a ' sound sound\n```\n\n```\nlines = ['what shall we do with the drunken sailor', 'shave his belly with a rusty razor', 'put him in a long boat till hes sober']\n{for} line {in} lines\n {for} i {in} {range} 1 {to} 3\n {print} line\n {print} 'early in the morning'\n {for} i {in} {range} 1 {to} 3\n {print} 'way hay and up she rises'\n {print} 'early in the morning'\n```\n"
+ 12:
+ example_code: "```\n_ actions = 'clap your hands', 'stomp your feet', 'shout Hurray!'\n_ {for} action {in} actions\n_ {for} i {in} {range} 1 {to} 2\n_ {print} 'if youre happy and you know it'\n_ {print} action\n_ {print} 'if youre happy and you know it and you really want to show it'\n_ {print} 'if youre happy and you know it'\n_ {print} action\n```\n"
+ story_text: "In this song we can make it even easier to program 'if you're happy and you know it, clap your hands'. Because we can put all of the actions in a variable, check it out:\n\n### Exercise\nCan you add the right amount of indentation to each line to make the song play correctly?\nHint: Not all lines need indentation.\n"
+ name: Sing a song!
+ description: Print a song
+ songs_2:
+ levels:
+ 16:
+ story_text: "### Exercise\nFinish the nursery rhyme!\n"
+ story_text_2: "### Exersice 2\nNow create your own code for the nursery rhyme 'The wheels on the bus' on the same way!\n"
+ example_code: "```\nnumber = ['one', 'two', 'three', 'four', 'five', 'six', 'seven', 'eight', 'nine', 'ten']\nobject = ['on his drum', 'on his shoe', 'on his knee', 'on his door', 'on his hive', 'on his sticks', 'up in heaven', 'on his gate', 'on his vine', 'once again']\n\n_\n {print} 'This old man'\n {print} 'He played ' _\n {print} 'He played knick-knack ' _\n {print} 'With a knick-knack paddywhack'\n {print} 'Give the dog a bone'\n {print} 'This old man came rolling home'\n {sleep} 8\n {clear}\n```\n"
+ example_code_2: "```\nobject = ['wheels', 'doors', _]\nmovement = [ 'round and round', 'open and shut', _]\n```\n"
+ 12:
+ example_code: "```\n{define} twinkle\n {print} 'Twinkle'\n {print} _\n\n{call} twinkle\n{print} 'Up above the world so high'\n{print} 'Like a diamond in the sky'\n{call} _\n```\n"
+ story_text: "Songs contain a lot of repetition. We can capture it with a function!\n### Exercise\nLook at the example code with the function. Fill out the two lines so the full song is printed.\n"
+ description: Sing a song 2
+ default_save_name: Song 2
+ name: Sing a song! 2
+ tic:
+ description: Play a game of Tic Tac Toe!
+ default_save_name: Tic
+ levels:
+ 16:
+ example_code: "```\n# Create a list called field\n_ = ['.', '.', '.', '.', '.', '.', '.', '.', '.']\n\n# Create a function that prints the field\n{define} print_field\n _\n {print} 'TIC TAC TOE'\n {print} field[1] field[2] field[3]\n _\n _\n\n# Call the function\n```\n"
+ story_text: "Let's program a game of tic-tac-toe!\n\n### Exercise\nIn this adventure we'll start with creating an empty field.\n\n***Create a list called field*** This list will be our playing field. This list is filled with 9 dots, since there are no x's and o's yet at the start of our game.\n\n***Create a function that prints the field*** Firstly, clear the screen so the old playing fields will be removed. Then we print the first line of our Tic Tac Toe field. This line constists of the first 3 spots in our list field.\nWe have already programmed this line for you. Now finish the field by printing spot 4, 5, and 6 on the second row and spot 7, 8 and 9 in the third row.\n\n***Call the function that prints the field*** Now call the function.\n
{call}
with the function's name to call it up! We don't have to type that block of code again.\n\nCheck out this example code of a game of Twister. The function 'turn' contains a block of code that chooses which limb should go where.\n\n### Exercise\nFinish this code by setting the 2 variables chosen_limb and chosen_color.\nThen, choose how many times you want to call the function to give the twister spinner a spin.\n\n### Exercise 2\nImprove your code by adding a variable called 'people'. Use the variable to give all the players their own command in the game.\nFor example: 'Ahmed, right hand on green' or 'Jessica, left foot on yellow'.\n"
+ example_code: "```\nsides = 'left', 'right'\nlimbs = 'hand', 'foot'\ncolors = 'red', 'blue', 'green', 'yellow'\n\n{define} turn\n chosen_side = sides {at} {random}\n chosen_limb = limbs _\n chosen_color = colors _\n {print} chosen_side ' ' chosen_limb ' on ' chosen_color\n\n{print} 'Lets play a game of Twister!'\n{for} i {in} {range} 1 {to} _\n {call} turn\n {sleep} 2\n```\n"
+ 13:
+ story_text: "Now that you've learned how to use functions, you'll learn how to use a function with an argument.\nAn **argument** is a variable that is used within a function. It is not used outside the function.\n\nFor example in this code we've programmed the first verse of the song 'My Bonnie is over the ocean'.\nIn this example code the argument 'place' is used. Place is a variable that is only used in the function, so an argument.\nTo use 'place' we have placed `{with} place` after `{define} song`.\nWhen the function is called, the computer will replace the argument 'place', with the piece of text after `{call} song {with}`.\n\n### Exercise\nThe next verse of this song goes:\n\n```not_hedy_code\nLast night as I lay on my pillow\nLast night as I lay on my bed\nLast night as I lay on my pillow\nI dreamed that my Bonnie is dead\n```\n\nCan you program this verse in the same way as the example?\n"
+ example_code: "```\n{define} song {with} place\n {print} 'My Bonnie is over the ' place\n\n{call} song {with} 'ocean'\n{call} song {with} 'sea'\n{call} song {with} 'ocean'\n```\n"
+ 18:
+ story_text: "Let's make functions the Pythons way! To define a function, we no longer use:\n\n`{define} name_function {with} argument_1, argument_2:`\n\nbut we use:\n\n`{def} name_function(argument_1, argument_2):`.\n\n\nIf you don't want to use arguments, you just leave the space between the parantheses empty.\nTo call a function, we don't need the `{call}` command anymore. You just type the name of the function.\n"
+ example_code: "```\n{def} calculate_score(answer, correct_answer):\n {if} answer == correct_answer:\n score = 1\n {elif} answer == '?':\n score = 0\n {else}:\n score = -1\n {return} score\n\nanswer = {input} ('Where can you find the Eiffel Tower?')\ncorrect_answer = 'Paris'\nscore = calculate_score(answer, correct_answer)\n{print} ('Your score is... ', score)\n```\n"
+ description: functions
+ default_save_name: functions
+ name: functions
+ dice:
+ levels:
+ 6:
+ story_text: "You can also make an Earthworm die again in this, but now you can also calculate how many points have been rolled.\nYou may know that the worm counts 5 points for Earthworms. Now after a roll you can immediately calculate how many points you have thrown.\nThis is the code to calculate points for one die:\n\n### Exercise\nCan you make the code so that you get the total score for 8 dice? To do that, you have to copy and paste some lines of the code.\n"
+ example_code: "```\nchoices = 1, 2, 3, 4, 5, earthworm\npoints = 0\nthrow = choices {at} {random}\n{print} 'you threw ' throw\n{if} throw {is} earthworm points = points + 5 {else} points = points + throw\n{print} 'those are ' points ' points'\n```\n"
+ example_code_2: "Did you manage to calculate the score for 8 dice? That required a lot of copy and pasting, right? We are going to make that easier in level 7!\n"
+ 7:
+ example_code: "```\nchoices = 1, 2, 3, 4, 5, 6\n_ _ _ _ _ _ _\n```\n"
+ story_text: "You can also make a dice again in this level. With the `{repeat}` code you can easily roll a whole hand of dice.\n\n### Exercise\nTry to finish the sample code! **Extra** Think of a game you know that involves a dice and program that using a `{repeat}`.\n"
+ 3:
+ story_text_2: "### Exercise\nThe dice in the example above are dice for a specific game. Can you make normal dice?\nOr other special dice from a different game?\n"
+ example_code_2: "```\nchoices {is} _\n```\n"
+ example_code: "```\nchoices {is} 1, 2, 3, 4, 5, earthworm\n{print} You threw _ {at} {random} !\n```\n"
+ story_text: "In this level we can choose from a list. With that we can let the computer choose one side of the die.\nTake a look at the games you have in your closet at home.\nAre there games with a (special) die? You can also copy it with this code.\nFor example, the dice of the game Earthworms with the numbers 1 to 5 and an earthworm on it.\n\n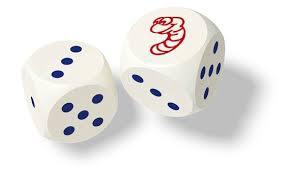\n"
+ 15:
+ example_code: "```\noptions = 1, 2, 3, 4, 5, 6\n{print} 'Throw 6 as fast as you can!'\nthrown = 0\ntries = 0\n_\n_\n_\n_\n_\n{print} 'Yes! You have thrown 6 in ' tries ' tries.'\n```\n"
+ story_text: "### Exercise\nIn this level you can create a little game in which you'll have to throw 6 as fast as possible.\nWe have started the code, it's up to you to get the game to work!\n\nFirstly, add a `{while}` loop that checks if 6 has been thrown or not.\nAs long as you haven't thrown 6 already, throw the dice on a random number.\nPrint what the player has thrown.\nAdd a try to the amount of tries\nWait a second before you throw again, or - in case you've thrown a 6 - before the game ends.\n"
+ 10:
+ story_text: "### Exercise\nIs everybody taking too long throwing the dice? In this level you can let Hedy throw all the dice at once!\nChange the names into names of your friends or family, and finish the code.\n"
+ example_code: "```\nplayers = Ann, John, Jesse\nchoices = 1, 2, 3, 4, 5, 6\n_ _ _ _\n {print} player ' throws ' choices {at} {random}\n {sleep}\n```\n"
+ 4:
+ story_text: "In this level you can also create dice. But this time you can try it yourself, without an example code!\n\n### Exercise\nMake your own dice in this level.\nTip: If you have no idea how to make dice. Take a peek at your dice from the previous level, but don't forget to add quotation marks.\n"
+ 5:
+ example_code: "```\nchoices {is} 1, 2, 3, 4, 5, earthworm\nthrow {is} choices {at} {random}\n{print} 'you have thrown ' throw\n_ throw {is} earthworm {print} 'You can stop throwing.'\n_ {print} 'You have to throw it again!'\n```\n"
+ story_text: "We are going to add the `{if}` and `{else}` commands to our dice!\n\n### Exercise\nComplete the sample code so that the code says \"You can stop throwing\" once you have thrown an earthworm. It should say \"You have to throw again\" if you've thrown anything else.\n**Extra** Maybe you want to recreate a die from a completely different game. That's fine too! Then make up your own reaction, e.g. 'yes' for 6 and 'pity' for something else.\n"
+ default_save_name: Dice
+ name: Dice
+ description: Make your own dice
+ while_command:
+ name: '{while}'
+ levels:
+ 15:
+ story_text: "We are going to learn a new loop, the `{while}` loop! We continue the loop as long as the statement is true.\nSo don't forget to change the value in the loop.\n\nIn the example code, we continue until a correct answer has been given.\nIf the correct answer is never given, the loop never ends!"
+ example_code: "```\nanswer = 0\n{while} answer != 25\n answer = {ask} 'What is 5 times 5?'\n{print} 'A correct answer has been given'\n```\n"
+ description: '{while}'
+ default_save_name: while_command
+ elif_command:
+ description: '{elif}'
+ levels:
+ 17:
+ story_text: "In this level you can also use a new command: `{elif}`. `{elif}` is a combination of the keywords `{else}` and `{if}` and you need it when you want to make 3 (or more!) options.\nCheck it out!\n"
+ example_code: "```\nprizes = ['1 million dollars', 'an apple pie', 'nothing']\nyour_prize = prizes[{random}]\n{print} 'You win ' your_prize\n{if} your_prize == '1 million dollars' :\n {print} 'Yeah! You are rich!'\n{elif} your_prize == 'an apple pie' :\n {print} 'Lovely, an apple pie!'\n{else}:\n {print} 'Better luck next time..'\n```\n"
+ name: '{elif}'
+ default_save_name: elif
+ music:
+ levels:
+ 7:
+ example_code: "```\n{print} 'Twinkle Twinkle Little Star'\n{repeat} 2 {times} {play} C4\n{repeat} 2 {times} {play} G4\n_\n```\n"
+ story_text: "Using the `{repeat}` command can make your codes for melodies a lot shorter!\n\n### Exercise\nFinish the code for Twinkle Twinkle Little Star by using the `{repeat}`command.\nThen go back to the songs you've made in the previous levels. Can you shorten those codes too?\n"
+ 6:
+ example_code: "```\nnumber = {ask} 'Say a starting number between 1 and 67'\n{print} number\n{play} number\nnumber = number + 1\n{print} number\n{play} number\nnumber = number + 1\n{print} number\n{play} number\n```\n"
+ story_text: "Instead of playing notes, you can also play numbers now. Simply type `{play} 1` for the lowest note, `{play} 70` for the highest note, or anything in between.\n\n### Exercise\nThis calls for musical maths! Try out the example code a couple of times with different starting numbers.\nThen, see if you can compose a song using the numbers.\n"
+ 9:
+ story_text: "From this level on you can - among other things - use a {repeat} command inside a {repeat} command.\nThat makes songs like 'Happy birthday' even shorter!\n\n### Exercise\nFinish the song!\n"
+ example_code: "```\nfirst_time = yes\n{repeat} 2 {times}\n {repeat} 2 {times}\n {play} C\n {play} D\n {play} C\n {if} first_time {is} yes\n {play} F\n {play} E\n first_time {is} no\n {else}\n _\n```\n"
+ 4:
+ example_code: "```\n{print} 'Mary had a little lamb'\n{play} E\n{play} D\n{play} C\n{play} D\n{play} E\n{play} E\n{play} E\n{clear}\n{print} 'Little lamb, little lamb'\n{play} D\n{play} D\n{play} D\n{play} E\n{play} E\n{play} E\n{clear}\n{print} 'Mary had a little lamb'\n{play} E\n```\n"
+ story_text: "Use the `{clear}` command to create a karaoke machine!\n\n### Exercise\nFinish the karaoke version of 'Mary had a little lamb'.\nThen, create a karaoke version of any song you'd like!\n"
+ 3:
+ example_code: "```\nnotes {is} A4, B4, C4\n{play} notes {at} {random}\n{play} notes {at} {random}\n{play} notes {at} {random}\n```\n"
+ story_text: "Create a random melody!\n\n### Exercise\nThe example code creates a random melody, but it's very short and not many notes are used.\nAdd more notes to the list and create a longer melody by copying the last line a couple more times.\n"
+ 13:
+ example_code: "```\n{print} 'Yankee Doodle'\n{define} _ {with} note_1, note_2, note_3\n {play} C4\n {play} C4\n {play} D4\n {play} E4\n {play} _\n {play} _\n {play} _\n\n{call} line_1 {with} 29, 31, 30\n{call} line_1 {with} 29, 28, 0\n{call} line_1 {with} 32, 31, 30\n\n{play} C4\n{play} B3\n{play} G3\n{play} A3\n{play} B3\n{play} C4\n{play} C4\n```\n"
+ story_text: "You can use a function with an argument for songs that have line that are almost the same, but slightly different each time.\nOne example is the song 'Yankee Doodle'. The first 4 notes of the first lines are the same, but each time they are followed by a different couple of notes.\n\n### Exercise\nCan you finish the song of Yankee Doodle?\nCan you think of another song to program this way?\n"
+ 5:
+ story_text: "You don't always have to use the `{play}` command to play a whole song, sometimes you just want to play one note.\nFor example, if you want to make a quiz, you can play a happy high note if the answer is right and a sad low note if the answer is wrong.\n\n### Exercise\nFinish the first question by adding a line of code that plays a C3 note if the wrong answer is given.\nThen think of 3 more questions to add to this quiz.\n"
+ example_code: "```\nanswer {is} {ask} 'What is the capital of Zimbabwe?'\n{if} answer {is} Harare {play} C6\n_\n```\n"
+ 2:
+ story_text_2: As you can see, you can also use the `{sleep}` command to add a little pause in the song.
+ example_code_2: "```\n{print} Twinkle Twinkle Little Star\n{play} C\n{play} C\n{play} G\n{play} G\n{play} A\n{play} A\n{play} G\n{sleep} 1\n{play} F\n{play} F\n```\n"
+ example_code: "```\n{print} Old Mac Donald had a farm\n{play} C5\n{play} C5\n{play} C5\n{play} G4\n{play} A4\n{play} A4\n{play} G4\n```\n"
+ story_text: "### Exercise\nFinish the songs! We have started the codes for some melodies.\n"
+ 1:
+ story_text: "In this level you'll learn how to use the `{play}` command to play a tune!\n\nType `{play}` followed by the note you want to play. The scale goes C-D-E-F-G-A-B.\nAs you can see there are 7 different letters, but we can play more than just 7 notes.\nType a number between 1 and 10 behind the letter to choose the scale, for example after B4 comes C5.\nC1 is the lowest note you can play, C10 is the highest.\n\n### Exercise\nTry out the example code and then play around with it! Can you create your own melody?\nIn the next level you'll learn how to play some existing songs."
+ example_code: "```\n{play} C4\n{play} D4\n{play} E4\n{play} F4\n{play} G4\n{play} A4\n{play} B4\n{play} C5\n```"
+ 14:
+ story_text: "You can program music for fun, but you can also use the musical notes to make something useful like a fire alarm!\n\n### Exercise\nMake sure the fire alarm rings when there is a fire!\n"
+ example_code: "```\n{define} fire_alarm\n {print} 'FIRE!'\n note = 40\n {for} i {in} {range} 1 {to} 100\n {if} note _ 50\n note = note + 5\n {play} _\n {else}\n note = 40\n\nfire = {ask} 'Is there a fire?'\n{if} fire _ 'yes'\n {call} fire_alarm\n```\n"
+ 17:
+ story_text: "You can use the {elif} to create different options.\n\n### Exercise\nFirstly, add colons to get the code to work.\nThen finish this code by adding at least 2 other songs for other moods. For example a happy song and an angry song.\n"
+ example_code: "```\n{define} scary_song\n {for} i {in} {range} 1 {to} 3\n {play} G\n {play} E\n {sleep} 2\n {for} i {in} {range} 1 {to} 3\n {play} F\n {play} D\n\nmood = {ask} 'Which emotion are you feeling?'\n{if} mood {is} 'fear'\n {call} scary_song\n{elif} _\n```\n"
+ 18:
+ story_text: "### Exercise\nEven in this last level of Hedy we can make some music! Be careful of all the syntax that is needed now.\nTake a good look at how the functions are defined and called upon in the example code.\nFinish the song!\n"
+ example_code: "```\n{def} line_1():\n {for} i {in} {range}(1, 5):\n {play} A\n {play} D\n {play} F\n {play} A\n\n{def} line_2():\n {for} i {in} {range}(1, 5):\n {play} G\n {play} C\n {play} E\n {play} G\n\n{def} line_3():\n_\n\n{print} ('The drunken sailor')\n{print} ('What shall we do with the drunken sailor?')\nline_1()\nline_2()\nline_3()\n{print} ('Early in the morning')\n```\n"
+ 15:
+ example_code: "```\n{define} song\n {play} _\n\nyes_or_no = {ask} 'Do you want to hear my never-ending song?'\n{while} yes_or_no = 'yes'\n {call} song\n {print} '🥳'\n```\n"
+ story_text: "**Warning** This adventure can become extremely annoying!\nWe can also use the {while} command to repeat a song forever.\n\n### Exercise\nFinish the never-ending song.\n"
+ 8:
+ example_code: "```\n{print} 'Brother John'\n{repeat} 2 {times}\n {play} C\n {play} D\n {play} E\n {play} C\n{repeat} 2 {times}\n {play} E\n {play} F\n {play} G\n {sleep} 1\n```\n"
+ story_text: "Now that we can use the `{repeat}` command for multiple lines, we can make songs even more easily!\n\n### Exercise\nFinish the song of Brother John (Frère Jacques). Don't forget to use `{repeat}`!\n"
+ 16:
+ story_text: "Upgrade your Old MacDonald code!\n\n### Exercise\nTake your code from the 'Sing a Song' adventure and add musical notes to it!\nYou can make a function for each line in the song and call that function after the line is printed.\nWe defined the first line for you and called it in the code. Can you finish the whole song?\n"
+ example_code: "```\n{define} line_1\n {for} i {in} {range} 1 {to} 3\n {play} G\n {play} D\n {for} i {in} {range} 1 {to} 2\n {play} E\n {play} D\n\nanimals = ['pig', 'dog', 'cow']\nsounds = ['oink', 'woof', 'moo']\nfor i in range 1 to 3\n animal = animals[i]\n sound = sounds[i]\n print 'Old MacDonald had a farm'\n call line_1\n print 'E I E I O!'\n _\n```\n"
+ 12:
+ story_text: "Use functions in your songs! As you can see in the example code, you can make a function for each line of Twinkle Twinkle Little Star. Once you've programmed the first three lines, all you have to do is call the functions in the order you want them played in.\n\n### Exercise\nFinish the song of Twinkle Twinkle Little Star.\nThen look back at all the songs you've programmed in the levels before, can you make those codes better and shorter using functions too?\n"
+ example_code: "```\n{define} first_line\n {play} C\n {play} C\n {play} G\n {play} G\n {play} A\n {play} A\n {play} G\n {sleep}\n\n{define} second_line\n {play} F\n {play} F\n {play} E\n {play} E\n {play} D\n {play} D\n {play} C\n {sleep}\n\n{define} third_line\n {play} G\n {play} G\n {play} F\n {play} F\n {play} E\n {play} E\n {play} D\n {sleep}\n\n{call} _\n{call} _\n{call} _\n{call} _\n{call} _\n{call} _\n```\n"
+ default_save_name: music
+ name: music
+ description: Play a tune!
+ for_command:
+ default_save_name: for
+ levels:
+ 18:
+ story_text: "Lastly, we'll turn `{for} i {in} {range} 1 to 5` into real Python code, like this:\n"
+ example_code: "```\n{for} i {in} {range}(1,5):\n {print} (i)\n```\n"
+ 10:
+ story_text_2: "### Exercise\nFinish this code by adding `{for} action {in} actions` to line 2.\n"
+ example_code_2: "```\nactions = clap your hands, stomp your feet, shout Hurray!\n_\n {repeat} 2 {times}\n {print} 'If youre happy and you know it, ' action\n {sleep} 2\n {print} 'If youre happy and you know it, and you really want to show it'\n {print} 'If youre happy and you know it, ' action\n {sleep} 3\n```\n"
+ example_code: "```\nanimals = dog, cat, blobfish\n{for} animal {in} animals\n {print} 'I love ' animal\n```\n"
+ story_text: "## For\nIn this level we learn a new code called `{for}`. With `{for}` you can make a list and use all elements.\n`{for}` creates a block, like `{repeat}` and `{if}` so all lines in the block need to start with 4 spaces."
+ 11:
+ example_code: "```\n{for} counter {in} {range} 1 {to} 10\n {print} counter\n{print} 'Ready or not. Here I come!'\n```\n"
+ story_text: "In this level, we add a new form of the `{for}`. In earlier levels, we used `{for}` with a list, but we can also use `{for}` with numbers.\nWe do that by adding a variable name, followed by `{in}` `{range}`. We then write the number to start at, `{to}` and the number to end at.\n\nTry the example to see what happens! In this level again, you will need to use indentations in lines below the `{for}` statements."
+ 17:
+ story_text: "Now we are going to change indentation a little bit. Every time that we need an indentation, we need `:` at the line before the indentation.\n"
+ example_code: "```\n{for} i {in} {range} 1 {to} 10:\n {print} i\n{print} 'Ready or not, here I come!'\n```\n"
+ name: '{for}'
+ description: '{for} command'
+ random_command:
+ levels:
+ 3:
+ story_text_3: "### Exercise\nTry out the `{at} {random}` command by making your own gameshow (like the ones on tv) where you choose a door or suitcase and it contains a big price!\nCan you do it? We have already put the first lines into the example code.\n"
+ story_text: "## At random\nIn this level you can make a list using the `{is}` command. You can let the computer choose a random item from that list. You do that with `{at} {random}`.\n"
+ example_code_2: "```\nfood {is} sandwich, slice of pizza, salad, burrito\n{print} I am going to have a food {at} {random} for lunch.\n```\n"
+ example_code: "```\nanimals {is} dogs, cats, kangaroos\n{print} animals {at} {random}\n```\n"
+ story_text_2: "You can use the `{at} {random}` command in a sentence as well.\n"
+ example_code_3: "```\n{print} The big gameshow!\n{print} There are 3 suitcases in front of you...\nchosen {is} {ask} Which suitcase do you choose?\nprices {is} _\n_\n```\n"
+ 16:
+ story_text: "We are going to make lists the Python way, with square brackets around the lists! We also keep the quotation marks around each item like we have learned in previous levels.\nWe use square brackets to point out a place in a list. For example: `friends[1]` is the first name on the list of friends, as you can see in the first part of the example code.\nThe second part of the example code shows you that we can also match 2 lists using the variable i."
+ story_text_2: "Now that you've learned to use the brackets in lists, you can also start using the {at} {random} command in the Python way!\nYou simply type the name of your list with `[random]` behind it!"
+ example_code: "```\nfriends = ['Ahmed', 'Ben', 'Cayden']\n{print} friends[1] ' is the first friend on the list.'\n{print} friends[2] ' is the second friend on the list.'\n{print} friends[3] ' is the third friend on the list.'\n#now we will match 2 lists using the variable i\nlucky_numbers = [15, 18, 6]\n{for} i {in} {range} 1 {to} 3\n {print} friends[i] 's lucky number is ' lucky_numbers[i]\n```\n"
+ example_code_2: "```\nfruit = ['apple', 'banana', 'cherry']\n{print} fruit[random]\n```"
+ name: '{random}'
+ default_save_name: random_command
+ description: introducing at random command
+ debugging:
+ levels:
+ 11:
+ story_text: "### Exercise\nDebug this calendar program. The output of this program is supposed to look like a list of dates.\nFor example:\n\n```\nHedy calendar\nHere are all the days of November\nNovember 1\nNovember 2\nNovember 3\n```\nAnd so on.\n\nMind that you have to test your code extra carefully for the month February, because the amount of days in this month changes in leap years."
+ example_code: "**Warning! This code needs to be debugged!**\n```\n{print} 'Hedy calendar'\nmonths_with_31 days = January, March, May, July, September, October, December\nmonths_with_30_days = April, June, August, November\nmonth = {ask} 'Which month would you like to see?'\n{if} month {in} months_with_31_days\n days = 31\n {if} month {in} months_with30_days\n days = 30\n{if} month = February\n leap_years = 2020, 2024, 2028, 2036, 2040, 2044, 2028\n year = {ask} 'What year is it?'\n{if} year {in} leap_years\n days = 29\n{else}\n days = 28\n\n{print} 'Here are all the days of ' moth\n{for} i {in} {range} 1 {to} days\n {print} month i\n```\n"
+ 15:
+ example_code: "**Warning! This code needs to be debugged!**\n```\nnames = 'Tanya', 'Romy', 'Kayla', 'Aldrin', 'Ali'\nverbs='walking', 'skipping', 'cycling', 'driving', 'running'\nlocations = 'on a mountaintop', 'in the supermarket', 'to the swimming pool'\nhiding_spots = 'behind a tree', under a table', in a box'\nsounds = 'a trumpet', 'a car crash', 'thunder'\ncauses_of_noise = 'a television', 'a kid with firecrackers', 'a magic elephant', 'a dream'\n\nchosen_ name = names {at} {random}\nchosen_verb = verbs {at} {random}\nchosen_location = 'locations {at} {random}'\nchosen_sounds = noises {at} {random}\nchosen_spot = hiding_spots {random}\nchosen_causes = causes_of_noise {at} {random}\n\n{print} chosen_name ' was ' chosen_verb ' ' chosen_location\n{print} 'when they suddenly heard a sound like ' sounds {at} {random}\n{print} chosen_name ' looked around, but they couldn't discover where the noise came from'\n{print} chosen_name ' hid ' chosen_spot'\n{print} 'They tried to look around, but couldn't see anything from there'\nhidden = 'yes'\n{while} hidden = 'yes'\n {print} chosen_name 'still didn't see anything'\nanswer = {ask} 'does ' chosen_name ' move from their hiding spot?'\n {if} answer = 'yes'\n hidden == 'no'\n{print} 'chosen_name moved from' chosen_spot\n{print} 'And then they saw it was just' chosen_cause\n{print} chosen_name 'laughed and went on with their day'\n{print} The End\n```\n"
+ story_text: "### Exercise\nDebug this random children's story. Good luck!"
+ 4:
+ story_text: "### Exercise\nDebug this code. Good luck!"
+ example_code: "**Warning! This code needs to be debugged!**\n```\n{print} 'Welcome to the online library!\n{ask} What genre of books do you like?\n{print} You like genre\nauthor {is} {ask} 'Who's your favorite author?'\n{print} 'author is your favorite author'\n{print} Hmmm... i think you should try... books {at} {random}\n```\n"
+ 17:
+ example_code: "**Warning! This code needs to be debugged!**\n```\n{define} food_order\n toppings = {ask} 'pepperoni, tuna, veggie or cheese?'\n size = {ask} 'big, medium or small?'\n number_of_pizza = {ask} 'How many these pizzas would you like?'\n\n {print} 'YOU ORDERED'\n {print} number_of_pizzas ' size ' topping ' pizza'\n\n{define} drinks_order\n drink = {ask} 'water, coke, icetea, lemonade or coffee?'\n number_of_drinks = {ask} 'How many of these drinks would you like?'\n\n {print} 'YOU ORDERED'\n {print} number_of_drinks ' ' drink\n\n'Welcome to Hedy pizza'\nmore_food = {ask} 'Would you like to order a pizza?'\n{while} more_food = 'yes'\n {return} food_order\n more_food = {ask} 'Would you like to order a pizza?'\nmore_drinks = {ask} 'Would you like to order some drinks?'\n{while} more_drinks == 'yes'\n {call} drink_order\n more_drinks == {ask} 'Would you like to order more drinks?'\n\n\n{print} 'Thanks for ordering!'\n```\n"
+ story_text: "### Exercise\nDebug this code. Good luck!"
+ 8:
+ example_code: "**Warning! This code needs to be debugged!**\n```\n{print} 'Welcome to Manicures and Pedicures by Hedy'\nbodypart = {ask} 'Are you getting your fingernails or toenails done today? Or both?'\n{if} bodyparts {is} both\n {print} That will be $25'\n price = 25\n {else}\n {print} That will be $18'\n price = 18\ncolor = {ask} What color would you like?\nsparkles = {ask} 'Would you like some sparkles with that?'\n{if} sparkles {is} yes\n {print} 'We charge $3 extra for that'\nprice = price + 3\n{else} {print} 'No sparkles' {print} 'So no extra charge'\n{sleep} 5\n{print} 'All done! That will be $' price ' please!'\n{print} 'Thank you! Byebye!'\n```"
+ story_text: "### Exercise\nDebug this code. Good luck!"
+ 10:
+ story_text: "### Exercise\nDebug this code. Good luck!"
+ example_code: "**Warning! This code needs to be debugged!**\n```\nnames = Muad Hasan Samira Noura\nactivities = fly a kite, go swimming, go hiking, catch tan in the sun\n{for} name {is} names\n{print} At the beach name loves to activity {at} {random}\n```\n"
+ 5:
+ example_code: "**Warning! This code needs to be debugged!**\n```\n{print} Welcome to Swimming Pool Hedy!\nclass {is} {ask} 'Are you here to join a class today?'\n{if} class yes\n{print} 'Great! You're joining a class!\n{print} {else} 'You will not be joining a class'\ndiscount {is} 'Do you have a discount code?'\n{if} discount {is} yes\ndiscount_answer {is} {ask} 'What's your discount code?'\ndiscount_codes = Senior4231, Student8786, NewMember6709\n{if} discount_answer {is} {in} discount_cods\n{print} 'That will be $3,50'\n'That will be $5,50'\n{print} 'Have a nice swim!'\n```\n"
+ story_text: "### Exercise\nDebug this code. Good luck!"
+ 2:
+ example_code: "**Warning! This code needs to be debugged!**\n```\ndestination {ask} Where are you going on holidays?\n{print} The flight to dstination leaves at 3 pm.\n{ask} Did you check in your luggage yet?\n{echo}\n{print} Let me print your boarding pass for you.\n{sleep}\nHere you go! Have a nice trip!\n```\n"
+ story_text: "Welcome to a debugging adventure. Debugging a code means getting rid of mistakes in the code.\nThat means that in these debugging adventures, we will give you a code that does not work yet.\nYou will have to figure out what's wrong and correct the mistakes.\n\n### Exercise\nDebug this code. Good luck!"
+ 9:
+ story_text: "### Exercise\nDebug this code. Good luck!"
+ example_code: "**Warning! This code needs to be debugged!**\n```\n{print} 'Welcome to our sandwich shop'\namount 'How many sandwiches would you like to buy?'\n{repeat} amount {times}\n{ask} {is} {ask} 'What kind or bread would you like your sandwich to be?'\ntypes_of_bread {is} white, wheat, rye, garlic, gluten free\n{if} chosen_bread in types_of_bread\n{print} 'Lovely!'\n{else}\n'I'm sorry we don't sell that'\ntopping {is} {ask} 'What kind of topping would you like?'\nsauce {is} {ask} 'What kind of sauce would you like?'\n{print} One chosen_bread with topping and sauce.\nprice = amount * 6\n{print} 'That will be 'price dollar' please'\n```\n"
+ 13:
+ story_text: "### Exercise\nDebug this code. Good luck!"
+ example_code: "**Warning! This code needs to be debugged!**\n```\n{define}movie_recommendation {with} name\n action_movies == 'Die Hard', 'Fast and Furious', 'Inglorious Bastards'\n romance_movies = 'Love Actually', 'The Notebook', 'Titanic'\n comedy_movies = 'Mr Bean' 'Barbie''Deadpool'\n kids_movies = 'Minions', 'Paddington', 'Encanto'\n {if} name {is} 'Camila' {or} name {is} 'Manuel'\n recommended_movie = kids_movie {at} {random}\n {if} name {is} 'Pedro' {or} 'Gabriella'\n mood = {ask} 'What you in the mood for?'\n {if} mood {is} 'action'\n recommended_movie = comedy_movies {at} {random}\n {if} mood {is} 'romance'\n recommended_movie = romance_movies\n {if} mood {is} 'comedy'\n recommended_movie = comedy_movies {at} {random}\n\n{print} 'I would recommend ' recommended_movie ' for ' name\n\nname = {ask} 'Who is watching?'\nrecommendation = {ask} 'Would you like a recommendation?'\n{if} recommendaion {is} 'yes'\n{print} movie_recommendation {with} name\n{else}\n{print} 'No problem!'\n```\n"
+ 14:
+ story_text: "### Exercise\nDebug this code. Good luck!"
+ example_code: "**Warning! This code needs to be debugged!**\n```\n{define} calculate_heartbeat\n {print} 'Press your fingertips gently against the side of your neck'\n {print} '(just under your jawline)'\n {print} 'Count the number of beats you feel for 15 seconds'\n beats == {ask} 'How many beats do you feel in 15 seconds?'\n heartbeat = beats*4\n {print} 'Your heartbeat is ' heartbeat\n {if} heartbeat >= 60 {or} heartbeat <= 100\n {print} 'Your heartbeat seems fine'\n {else}\n {if} heartbeat > 60\n {print} 'Your heartbeat seems to be too low'\n {if} heartbeat < 100\n {print} 'Your heartbeat seems to be too high'\n {print} 'You might want to contact a medical professional'\n\nmeasure_heartbeat = {ask} 'Would you like to measure your heartbeat?'\n{if} measure_heartbeat = 'yes'\n {call} measure_heartbeat\n{else}\n 'no problem'\n```\n"
+ 16:
+ story_text: "### Exercise\nDebug this code. Good luck!\nTip: Make sure that you only see your score once in the end."
+ example_code: "**Warning! This code needs to be debugged!**\n```\ncountry = ['The Netherlands', 'Poland', 'Turkey', 'Zimbabwe', 'Thailand', 'Brasil', 'Peru', 'Australia', 'India', 'Romania' ]\ncapitals = 'Amsterdam', 'Warshaw' 'Istanbul', 'Harare', 'Bangkok', 'Brasilia', 'Lima', 'Canberra', 'New Delhi', 'Bucharest'\nscore = 0\n{for} i {in} {range} 0 {to} 10\n answer = {ask} 'What's the capital of ' countries[i]\n correct = capital[i]\n {if} answer = correct\n {print} 'Correct!'\n score = score + 1\n {else}\n {print} 'Wrong,' capitals[i] 'in the capital of' countries[i]\n {print} 'You scored ' score ' out of 10'\n\n```\n"
+ 12:
+ story_text: "### Exercise\nDebug this code. Good luck!"
+ example_code: "**Warning! This code needs to be debugged!**\n```\n{define} greet\ngreetings = 'Hello', 'Hi there', 'Goodevening'\n {print} greetings {at} {random}\n\n{define} take_order\n food = {ask} 'What would you like to eat?'\n {print} 'One food'\n drink = 'What would you like to drink?'\n {print} 'One ' drink\n more = {ask} 'Would you like anything else?'\n {if} more {is} 'no'\n {print} 'Alright'\n {else}\n {print} 'And ' more\n{print} 'Thank you'\n\n{print} 'Welcome to our restaurant'\npeople = {ask} 'How many people are in your party tonight?'\n{for} i {in} {range} 0 {to} people\n {call} greet_costumer\n```\n"
+ 1:
+ story_text: "Welcome to a debugging adventure. Debugging a code means getting rid of mistakes in the code.\nThat means that in these debugging adventures, we will show you code that does not work yet.\nYou will have to figure out what's wrong and correct the mistakes.\n\n### Exercise\nDebug this code. Good luck!"
+ example_code: "**Warning! This code needs to be debugged!**\n```\n{print} I love programming\nDo you love programming too?\n{echo}\n{print} What are your hobbies?\n{echo} Your hobbies are\n```\n"
+ 18:
+ story_text: "### Exercise\nDebug this Old MacDonald program from level 16. Good luck!"
+ example_code: "**Warning! This code needs to be debugged!**\n```\nanimals = ['pig', 'dog', 'cow']\nsounds = ['oink', 'woof', 'moo']\n{for} i {in} {range} 1 {to} 3\n animal = animals[i]\n sound = sounds[i]\n {print} 'Old MacDonald had a farm'\n {print} 'E I E I O!'\n {print} 'and on that farm he had a ' animal\n {print} 'E I E I O!'\n {print} 'with a ' sound sound ' here'\n {print} 'and a ' sound sound ' there'\n {print} 'here a ' sound\n {print} 'there a ' sound\n {print} 'everywhere a ' sound sound\n```\n"
+ 7:
+ example_code: "**Warning! This code needs to be debugged!**\n```\nAre you sleeping?\nBrother John!\nMorning bells are ringing!\nDing, dang, dong!\n```\n"
+ story_text: "### Exercise\nSurprise! This program looks more like an output than a code. And yet, we don't want you to just add `{print}` commands in front of each line.\nFix this program to turn it into the nursery rhyme 'Brother John (Frère Jacques)' by using the {repeat} command of course!"
+ 3:
+ example_code: "**Warning! This code needs to be debugged!**\n```\nmovie_choices {is} dracula, fast and furious, home alone, barbie\nchosen_movie {is} movies {at} {random}\n{print} Tonight we will watch chosen _movies\nlike {ask} Do you like that movie?\n{print} Tomorrow we will watch something else.\n{add} chosen_movie {to_list} movie_choices\n{print} Tomorrow we will watch tomorrows_movie\ntomorrows_movie {is} movie_choices {at} {random}\nI'll go get the popcorn! {print}\n```\n"
+ story_text: "Welcome to a debugging adventure. Debugging a code means getting rid of mistakes in the code.\nThat means that in these debugging adventures, we will give you a code that does not work yet.\nYou will have to figure out what's wrong and correct the mistakes.\n\n### Exercise\nDebug this code. Good luck!"
+ 6:
+ example_code: "**Warning! This code needs to be debugged!**\n```\n{print} 'Vending machine'\nchosen_product = {ask} 'Please select a product'\n1_dollar_products = coke orange juice water\n2_dollar_products = chocolate, cookie, museli bar\n3dollar_prodcuts = potato chips, beef jerky, banana bread\n{if} chosen {is} {in} 1_dollar_products\nprice = 1\n{if} chosen_product {is} 2_dollar_products\nprice = 2\n{else} chosen_product {in} 3_dollar_products\nprice = 3\namount_of_products = '{ask} How many of ' chosen_product would you like to have?'\ntotal = price + amount_of_product\n{print} 'That will be $' price 'please'\n```\n"
+ story_text: "### Exercise\nDebug this code. Good luck!"
+ default_save_name: debugging
+ description: debugging adventure
+ name: debugging
+ rock:
+ levels:
+ 2:
+ story_text: "In this level you can practise using the variables, so that you can make the rock, paper, scissors game in the next level!\n### Exercise\nFinish the code by filling in the **variable** on the blanks.\nThis game is not very interactive, but no worries! In the next tab you'll learn how to use variables with the `{ask}` command to make your game interactive!\n"
+ example_code: "```\nchoice {is} rock\n{print} I choose _\n```"
+ 1:
+ example_code_2: "```\n{print} what do you choose?\n{ask} choose from _\n{echo} so your choice was:\n```\n"
+ story_text_2: "### Exercise\nInstead of using words, you could also use emojis: ✊✋✌\nCan you create a code using emojis?\n"
+ example_code: "```\n{print} what do you choose?\n{ask} choose from rock, paper or scissors\n{echo} so your choice was:\n```\n"
+ story_text: "In level 1 you can start with a rock, paper, scissors game.\n\nWith `{ask}` you can make a choice, and with `{echo}` you can repeat that choice.\n"
+ 3:
+ example_code_2: "```\nchoices {is} rock, paper, scissors\nplayer_1 {is} {ask} Name of player 1:\n_\n```\n"
+ story_text: "You can use the `{at} {random}` command to let the computer pick rock, paper or scissors!\n\n### Exercise\nFinish the code by using the `{at} {random}` command.\n"
+ story_text_2: "**Extra** Make a two player game. Firstly ask the two players to fill in their names. Then let the computer randomly pick their choices.\n"
+ example_code: "```\nchoices {is} rock, paper, scissors\n{print} choices _\n```\n"
+ 15:
+ story_text: "### Exercise\nPlay until you beat the computer! But first, finish the example code...\n"
+ example_code: "```\nwon = 'no'\noptions = 'rock', 'paper', 'scissors'\n{while} won == 'no'\n your_choice = {ask} 'What do you choose?'\n computer_choice = options {at} {random}\n {print} 'you chose ' your_choice\n {print} 'the computer chose ' computer_choice\n {if} computer_choice == your_choice\n {print} 'Tie!'\n {if} computer_choice == 'rock' {and} your_choice == 'scissors'\n {print} 'You lose!'\n {if} computer_choice == 'rock' {and} your_choice == 'paper'\n {print} 'You win!'\n won = 'yes'\n_\n```\n"
+ 10:
+ example_code: "```\nchoices = _\nplayers = _\n{for} _\n```\n"
+ story_text: "### Exercise\nIn the previous levels you have often made your own rock paper scissors game. Can you finish the code and use the `{for}` command properly to get the game to work?\n"
+ 5:
+ story_text: "In this level we can determine whether it's a tie or not. For that you need the new `{if}` code.\n\n### Exercise\nFinish the code by filling in the blanks:\n* Let the computer pick a random option\n* Ask the player what they want to choose\n* Fill in the correct variables in line 4 and 5\n* Finish line 6 so that Hedy can check whether it's a tie or not.\n"
+ example_code: "```\noptions {is} rock, paper, scissors\ncomputer_choice {is} _\nchoice {is} _\n{print} 'you chose ' _\n{print} 'computer chose ' _\n{if} _ {is} _ {print} 'tie!' {else} {print} 'no tie'\n```\n\nFill in the correct code on the blanks to see if it is a draw.\n"
+ 9:
+ example_code: "```\nchoices = rock, paper, scissors\nyour_choice {is} {ask} 'What do you choose?'\n{print} 'You choose ' your_choice\ncomputer_choice {is} choices {at} {random}\n{print} 'The computer chooses ' computer_choice\n{if} computer_choice {is} your_choice\n {print} 'Tie'\n{if} computer_choice {is} rock\n {if} your_choice {is} paper\n {print} 'You win!'\n {if} your_choice {is} scissors\n {print} 'You lose!'\n# finish this code\n```\n"
+ story_text: "In this level you can program the whole rock, paper, scissors game by nesting the `{if}` commands. \n\n### Exercise\nCan you finish the code? The program must tell who has won for every combination.\n\n**Extra** Want to play more than one game? Expand the code so that you can play multiple rounds. You can even use an `{ask}` to ask the user how many rounds they want to play.\n"
+ 13:
+ story_text: "With the `{and}` command you can shorten your rock, paper, scissors code! Check out the example code.\n\n### Exercise\nFinish the code such that a winner is always decided on. Run your code a few times to verify there is always a winner printed.\n"
+ example_code: "```\noptions = 'rock', 'paper', 'scissors'\nyour_choice = {ask} 'What do you choose?'\ncomputer_choice = options {at} {random}\n{print} 'You choose ' your_choice\n{print} 'The computer chooses ' computer_choice\n{if} computer_choice {is} your_choice\n {print} 'Tie'\n{if} computer_choice {is} 'rock' {and} your_choice {is} 'paper'\n {print} 'You win!'\n{if} computer_choice {is} 'rock' {and} your_choice {is} 'scissors'\n {print} 'The computer wins!'\n_\n```\n"
+ 4:
+ story_text: "In this level we can further program rock, paper, scissors. But if you want to add text, you have to use quotation marks here too.\n### Exercise\nFill in quotation marks on the blanks. Mind that the variable `choices` should be outside the quotes.\n"
+ example_code: "```\nchoices {is} rock, paper, scissors\n{print} _The computer chooses..._ choices {at} {random}\n```\n"
+ name: Rock, paper, scissors
+ default_save_name: Rock_2
+ description: Make your own rock, paper, scissors game
+ calculator:
+ levels:
+ 14:
+ example_code: "```\n{define} calculate_mean_grade\n total = 0\n {for} i {in} {range} 1 {to} 4\n grade = {ask} _\n total = total + _\n {return} _ / 4\n\nmean_grade = {call} _\n{print} 'Your mean grade is ' mean_grade\n```\n"
+ story_text: "In this adventure you will build a calculator that calculates your mean grade for you. If you get your calculator to work, you can move on to the next adventure, which allows you to add two extra features.\n\n### Exercise 1\nFill in the blanks to get the calculator to work.\n* Start with the fourth line, add a question to figure out what grade the student got.\n* In the fifth line you'll want to calculate the total of all grades, so the total = total + grade.\n* Then we get to set the return value. We want to return the mean, so the total devided by the amount of tests (4).\n* Lastly we finish the code by calling the function in line 8.\n\nDid you get it? Awesome! Would you like to add even more to your calculator? **This adventure continues in the next tab!**\n"
+ 9:
+ story_text: "In a previous level, you've created a calculator. In this level, you can expand that code so it asks multiple questions.\n\n### Exercise 1\nCan you finish line 10 to get the code to work?\n\n### Exercise 2\nGive the player feedback when they enter an answer, like `{print} 'Correct!'` or `{print} 'Wrong! The correct answer is ' correct_answer`.\n"
+ example_code: "```\nscore = 0\n{repeat} 10 {times}\n numbers = 1, 2, 3, 4, 5, 6, 7, 8, 9, 10\n number_1 = numbers {at} {random}\n number_2 = numbers {at} {random}\n correct_answer = number_1 * number_2\n {print} 'What is ' number_1 ' times ' number_2 '?'\n answer = {ask} 'Type your answer here...'\n {print} 'Your answer is ' answer\n {if} _ {is} _\n score = score + 1\n{print} 'Great job! Your score is... ' score ' out of 10!'\n```\n"
+ 10:
+ example_code: "```\nnumbers = 1, 2, 3\n{for} _\n {for} _\n answer = {ask} _\n correct = number_1 * number_2\n {if} answer {is} correct\n {print} 'Great job!'\n {else}\n {print} 'That is wrong. The right answer is ' correct\n```\n"
+ story_text: "This calculator game helps you practise your tables of multiplication!\n### Exercise\nFill in the blanks. We want this program to ask the player these questions:\n```\nHow much is 1 times 1?\nHow much is 1 times 2?\nHow much is 1 times 3?\nHow much is 2 times 1?\nHow much is 2 times 2?\nHow much is 2 times 3?\nHow much is 3 times 1?\nHow much is 3 times 2?\nHow much is 3 times 3?\n_\n```\n"
+ 13:
+ story_text: "### Exercise 1\nLet's make the practice program a bit harder. The player now has to answers two questions correctly. Fill out the blanks to complete the program.\n\n### Exercise 2\nSometimes, calculations have multiple correct answers. For example, 10 can be divided by 5 and by 2. So the question 'What number divides 10?' can be answered by 2 and by 5.\nAsk for a calculation that has multiple correct answers, ask the player to answer it, and determine if it is correct using `{or}`.\nEmpty the programming field and create your own solution.\n"
+ example_code: "```\nanswer1 = {ask} 'What is 10 times 7?'\nanswer2 = {ask} 'What is 6 times 7?'\n{if} _ _ _ _ _ _ _\n {print} _\n```\n"
+ 12:
+ story_text: "In this level, you can make a calculator that works for decimal numbers.\n\n### Exercise 1\nFill out the blanks to complete the calculator. Remember to use a period and not a comma for decimal numbers.\n\n### Exercise 2\nCreate a new mathematics practice program, but now use decimal numbers.\nCreate a list of numbers, choose two to multiple and let the player answer.\nAnd of course you have to validate the answer! **Extra** Increase the difficulty by adding lives: A player loses a life for a wrong answer and after three wrong answers the game ends.\n"
+ example_code: "```\nnumber1 = {ask} 'What is the first number?'\nnumber2 = {ask} 'What is the second number?'\nanswer = _\n{print} number1 ' plus ' number2 ' is ' _\n```\n"
+ 6:
+ story_text_3: "**Extra** You can also let the computer do random products on its own using `{random}`.\n"
+ example_code_3: "```\nnumbers = 1, 2, 3, 4, 5, 6, 7, 8, 9, 10\nnumber_1 = _\nnumber_2 = _\ncorrect_answer = number_1 * number_2\ngiven_answer = {ask} 'What is ' number_1 ' times ' number_2 '?'\n{if} _\n{else} _\n```"
+ story_text_2: "### Exercise\nThe calculator above will calculate the answer for you, but you can also make a program to test your own maths skills, like this:\nFill in the blanks to make it complete!\n"
+ story_text: "Now that you can do maths, you can make a calculator yourself!\n"
+ example_code: "```\nnumber_1 = {ask} 'Fill in the first number:'\nnumber_2 = {ask} 'Fill in the second number:'\ncorrect_answer = number_1 * number_2\n{print} number_1 ' times ' number_2 ' is ' correct_answer\n```\n"
+ example_code_2: "```\ncorrect_answer = 11 * 27\nanswer = {ask} 'How much is 11 times 27?'\n{if} answer {is} _ {print} 'good job!'\n{else} {print} 'Wrong! It was ' _\n```\n"
+ 15:
+ example_code: "```\n{define} new_question\n numbers = 1, 2, 3, 4, 5, 6, 7, 8, 9, 10\n number_1 = numbers {at} {random}\n number_2 = numbers {at} {random}\n correct = number_1 * number_2\n answer = 0\n _\n _\n _\n {print} 'Well done!'\n\n{print} 'Give 10 correct answers to win!'\n{for} i {in} {range} 1 {to} 10\n _\n{print} 'You win!'\n```\n"
+ story_text: "You can add the `{while}` loop to the calculator game you've learned to make in a previous level.\nThis makes sure the player can't continue to the next question if they answer incorrectly.\n\n### Exercise\nAdd the `{while}` loop in the function, ask the player what number_1 times number_2 is and print their answer.\nThen `{call}` the function.\n"
+ 11:
+ story_text: "With a `{for}` you can simplify tables of multiplication practise program.\n\n### Exercise 1\nImprove the example code such that it prints a nice multiplication table: >>=y=v>>>24,p-=y,!(16&(y=v>>>16&255))){if(0==(64&y)){v=_[(65535&v)+(d&(1<
\").replace(/\\r?\\n/g,\"
\").replace(/\\t/g,\" \").replace(/^\\s/,\" \").replace(/\\s$/,\" \").replace(/\\s\\s/g,\" \");if(t.includes(\"
\")||t.includes(\"
\")){t=`
${t}
`}return t}function SL(t){return t.replace(/(\\s+)<\\/span>/g,((t,e)=>{if(e.length==1){return\" \"}return e})).replace(//g,\"\")}const BL=[\"figcaption\",\"li\"];const ML=[\"ol\",\"ul\"];function PL(t){if(t.is(\"$text\")||t.is(\"$textProxy\")){return t.data}if(t.is(\"element\",\"img\")&&t.hasAttribute(\"alt\")){return t.getAttribute(\"alt\")}if(t.is(\"element\",\"br\")){return\"\\n\"}let e=\"\";let n=null;for(const o of t.getChildren()){e+=NL(o,n)+PL(o);n=o}return e}function NL(t,e){if(!e){return\"\"}if(t.is(\"element\",\"li\")&&!t.isEmpty&&t.getChild(0).is(\"containerElement\")){return\"\\n\\n\"}if(ML.includes(t.name)&&ML.includes(e.name)){return\"\\n\\n\"}if(!t.is(\"containerElement\")&&!e.is(\"containerElement\")){return\"\"}if(BL.includes(t.name)||BL.includes(e.name)){return\"\\n\"}return\"\\n\\n\"}function LL(t,e){return t&&hl(t,e,Gi)}const zL=LL;var OL=1,RL=2;function VL(t,e,n,o){var i=n.length,r=i,s=!o;if(t==null){return!r}t=Object(t);while(i--){var a=n[i];if(s&&a[2]?a[1]!==t[a[0]]:!(a[0]in t)){return false}}while(++i node\n if (codeNode) {\n codeNode.hidden = true\n code = codeNode.innerText\n } else {\n code = preview.textContent || \"\";\n preview.textContent = \"\";\n }\n\n // Create this example editor\n const exampleEditor = editorCreator.initializeReadOnlyEditor(preview, dir);\n // Strip trailing newline, it renders better\n exampleEditor.contents = code;\n exampleEditor.contents = exampleEditor.contents.trimEnd();\n // And add an overlay button to the editor if requested via a show-copy-button class, either\n // on the itself OR on the element that has the '.turn-pre-into-ace' class.\n if ($(preview).hasClass('show-copy-button') || $(container).hasClass('show-copy-button')) {\n const buttonContainer = $('').addClass('absolute ltr:right-0 rtl:left-0 top-0 mx-1 mt-1').appendTo(preview);\n let symbol = \"\u21E5\";\n if (dir === \"rtl\") {\n symbol = \"\u21E4\";\n }\n const adventure = container.getAttribute('data-tabtarget')\n $('